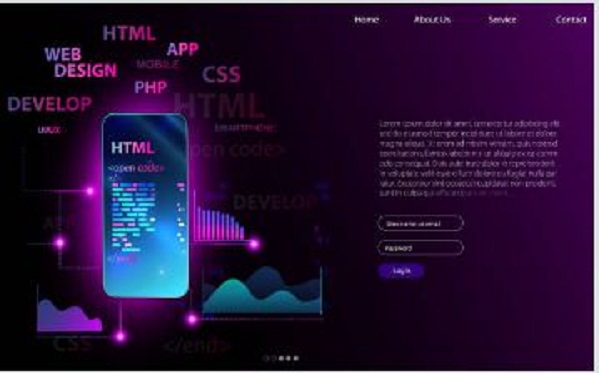
January 27, 2023, 2 Comments
Can you run a matlab script in c++?
MATLAB (Matrix Laboratory) is a programming language and platform that is widely used for mathematical and scientific computing. On the other hand, C is a general-purpose programming language that is widely used for system programming, embedded systems, and other low-level tasks. Can you run a Matlab script in c++? It is possible to run a Matlab script in c++, but it requires some additional steps and setup.
Calling MATLAB Functions from C
One way to run a MATLAB script in c++ is to call MATLAB functions from C code. This can be done using the MATLAB Engine API for C, which provides a set of functions that allow C programs to interact with the MATLAB runtime. The MATLAB Engine API for C is available as a library that can be linked to C programs, and it allows C programs to call MATLAB functions, create and manipulate MATLAB arrays, and exchange data with the MATLAB workspace.
Compiling a MATLAB Script into a C Shared Library
Another way to run a MATLAB script in c++ is to compile the MATLAB script into a C shared library. This can be done using the MATLAB Compiler SDK, which is a tool that allows you to create standalone executables and C shared libraries from MATLAB code. The MATLAB Compiler SDK generates C code from the MATLAB code and then compiles the C code into a shared library that can be linked to C programs.
The MATLAB Compiler SDK also includes a C header file that contains the prototypes for the functions in the shared library, so that C programs can call the functions in the shared library just like any other C function.
Limitations
It should be noted that both of the above methods have some limitations. The MATLAB Engine API for C is only available for Windows and Linux. And it requires that MATLAB install on the system. The MATLAB Compiler SDK is not free and requires a license. Additionally, the performance of the generated code may not be as good as native C code, and the generated code may have additional dependencies on MATLAB libraries.
Using the MATLAB Engine API for C
To use the MATLAB Engine API for C, you first need to install the MATLAB Engine API for C library on your system. The library includes MATLAB and can found in the matlabroot/extern/lib/arch folder, where Matlab root is the root folder of the MATLAB installation.
Once the library is installed. You can include the header file “matlabengine.h” in your C code and link to the library to use the MATLAB Engine API for C functions. The following is an example of how to use the MATLAB Engine API for C to call a MATLAB function from C:
Copy code
#include <stdio.h>
#include <matlabengine.h>int main()
{
Engine *ep;
mxArray *result = NULL;
double data[] = {1, 2, 3, 4, 5};
mxArray *input;/* Start the MATLAB engine */
if (!(ep = engOpen(“”))) {
fprintf(stderr, “\nCan’t start MATLAB engine\n”);
return EXIT_FAILURE;
}
/* Create a 1-by-5 mxArray from data */
input = mxCreateDoubleMatrix(1, 5, mxREAL);
memcpy((void *)mxGetPr(input), (void *)data, sizeof(data));
/* Call the MATLAB function */
engPutVariable(ep, “input”, input);
engEvalString(ep, “result = mean(input);”);
result = engGetVariable(ep, “result”);
/* Print the result */
printf(“The mean value is: %f\n”, *mxGetPr(result));
/* Clean up */
mxDestroyArray(input);
mxDestroyArray(result);
engClose(ep);
return EXIT_SUCCESS;
}
This example starts the MATLAB engine, creates a mxArray from a double array, puts the mxArray into the MATLAB workspace as a variable called “input”, evaluates a MATLAB command to call the mean function on the input variable, retrieves the result variable from the MATLAB workspace, and prints the result.
Compiling a MATLAB Script into a C Shared Library
To use the MATLAB (Matrix Laboratory) Compiler SDK to create a C shared library from a MATLAB script, you first need to install the MATLAB Compiler SDK on your system. Once the MATLAB Compiler SDK is installed. You can use the MCC command to create a C shared library from a MATLAB script.
For example, if you have a MATLAB script called “myscript.m”, you can create a C shared library from the script with the following command:
Copy code
mcc -v -W csharedlib:myscript -T link:lib myscript.m
This command creates a C shared library called “myscript.dll” on Windows and “myscript.so” on Linux systems. The library contains the functions defined in the script, as well as any necessary MATLAB runtime libraries.
You can then include the header file “myscript.h” in your C code and link to the library to call the functions defined in the MATLAB script. The following is an example of how to call a function “myfunction” defined in the script
from C:
Copy code
#include <stdio.h>
#include “myscript.h”int main()
{
double result;
double data[] = {1, 2, 3, 4, 5};/* Call the function from the shared library */
result = myfunction(data, 5);
/* Print the result */
printf(“The result is: %f\n”, result);
return 0;
}
This example calls the “myfunction” function from the shared library and passes the data array as an argument, and stores the result in the “result” variable. Then it prints the result to the console.
When using the MATLAB Compiler SDK, the generated code may not be as efficient as hand-written C code and may have additional dependencies on MATLAB libraries. However, it can be a useful tool for quickly integrating MATLAB functionality into C programs. Also, can use to deploy MATLAB algorithms as standalone executables or C shared libraries.
It should be noted that the MATLAB Compiler SDK is a commercial product and requires a license to use. In addition to this, not all MATLAB functions or toolboxes are supported by the MATLAB Compiler SDK. So it is important to check the documentation to see if the functions you need supported
Conclusion
Can you run a Matlab script in c++, people also ask such questions. While it is possible to run a MATLAB script in C, it requires some additional steps and setup. The MATLAB Engine API for C can use to call MATLAB functions from C code. The MATLAB Compiler SDK can be used to compile a MATLAB script into a C-shared library. Both methods have some limitations and may not be suitable for all situations. In case of performance constraints, it recommends to re-implement the MATLAB script in c++.
Error 503 Backend Fetch Failed; Causes and Solutions - HS Marketing
February 14, 2023[…] systems, be it Windows, Mac or Linux are very intricate and consist of countless components that work together as one unit. It’s almost impossible to grasp the full complexity of a […]
How to Resolve Paramount Plus error code 4200? - HS Marketing
February 16, 2023[…] Many users have reported experiencing playback issues when streaming on various platforms. The most commonly reported buffering issue is a blank screen while attempting to watch content. To fix this problem, people are in search of possible solutions. […]